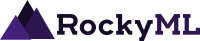 |
RockyML
0.0.1
A High-Performance Scientific Computing Framework
|
5 #ifndef ROCKY_BENCHMARK_GUARD
6 #define ROCKY_BENCHMARK_GUARD
7 #define _USE_MATH_DEFINES
22 #include<rocky/zagros/system.h>
23 #include<rocky/utils.h>
34 template<
typename T_e>
42 virtual T_e objective(T_e* x){
44 for(
size_t i=0; i<dim_; i++)
50 virtual std::string to_string(){
51 std::stringstream name;
52 name <<
"Sphere(dim=" << dim_ <<
")";
61 template<
typename T_e>
70 virtual T_e objective(T_e* x){
72 for(
size_t i=0; i<dim_-1; i++)
73 S += 100.0 * pow(x[i+1] - pow(x[i], 2), 2) + pow(1 - x[i], 2);
78 virtual std::string to_string(){
79 std::stringstream name;
80 name <<
"Rosenbrock(dim=" << dim_ <<
")";
85 template<
typename T_e>
101 virtual T_e objective(T_e* x){
103 for(
size_t i=0; i<dim_; i++)
104 S += (x[i]-shift_) * (x[i]-shift_) - 10.0*cos(2*M_PI * (x[i]-shift_));
109 virtual std::string to_string(){
110 std::stringstream name;
111 name <<
"Rastrigin(dim=" << dim_ <<
")";
116 template<
typename T_e>
132 virtual T_e objective(T_e* x){
134 tbb::combinable<T_e> partial_sums{0.0};
135 tbb::parallel_for(0, dim_, [&](
auto i){
136 partial_sums.local() += (x[i]-shift_) * (x[i]-shift_) - 10.0*cos(2*M_PI * (x[i]-shift_));
138 S += partial_sums.combine([](
auto x,
auto y){
return x+y; });
143 virtual std::string to_string(){
144 std::stringstream name;
145 name <<
"Parallel Rastrigin(dim=" << dim_ <<
")";
150 template<
typename T_e>
159 virtual T_e objective(T_e* x){
160 T_e S = 20.0 + exp(1.0);
163 for(
size_t i=0; i<dim_; i++){
165 S_c += cos(2*M_PI * x[i]);
169 S_s = -0.20 * sqrt(S_s);
170 S_s = -20.0 * exp(S_s);
178 virtual std::string to_string(){
179 std::stringstream name;
180 name <<
"Ackley(dim=" << dim_ <<
")";
189 template<
typename T_e>
209 virtual T_e objective(T_e* x){
213 for(
size_t i=0; i<dim_; i++){
214 S_p += pow(x[i], 2)/4000.0;
215 P_c *= cos(x[i]/sqrt(i+1));
222 virtual std::string to_string(){
223 std::stringstream name;
224 name <<
"griewank(dim=" << dim_ <<
")";
234 template<
typename T_e>
250 virtual T_e objective(T_e* x){
252 for(
size_t i=0; i<dim_; i++)
254 T_e value = -(1 + cos(12.0 * sqrt(S_s)))/(0.5 * S_s + 1);
259 virtual std::string to_string(){
260 std::stringstream name;
261 name <<
"dropwave(dim=" << dim_ <<
")";
268 template<
typename T_e>
275 std::mt19937 local_rng(0);
278 std::uniform_real_distribution<T_e> dist(-10.0, 10.0);
279 for(
int i=0; i<m; i++)
280 b_[i] = dist(local_rng);
282 for(
int i=0; i<m*n; i++)
283 A_[i] = dist(local_rng);
287 template<
typename T_e>
292 tbb::enumerable_thread_specific<thread_safe_least_squares<T_e>> problem_;
299 virtual T_e objective(T_e* x_){
300 T_e* A_ = problem_.local().A_.data();
301 T_e* b_ = problem_.local().b_.data();
303 Eigen::Map<Eigen::Matrix<T_e, Eigen::Dynamic, Eigen::Dynamic, Eigen::RowMajor>> A(A_, m_, n_);
304 Eigen::Map<Eigen::Matrix<T_e, Eigen::Dynamic, Eigen::Dynamic, Eigen::RowMajor>> b(b_, m_, 1);
305 Eigen::Map<Eigen::Matrix<T_e, Eigen::Dynamic, Eigen::Dynamic, Eigen::RowMajor>> x(x_, n_, 1);
307 auto error = (A*x - b).norm();
griewank(int dim=2, T_e lb=-20.0, T_e ub=20.0)
Construct a new griewank object.
Definition: benchmark.h:204
virtual T_e upper_bound()
upper bound specification should be used when upper bound is same for all parameters
Definition: benchmark.h:177
virtual T_e upper_bound()
upper bound specification should be used when upper bound is same for all parameters
Definition: benchmark.h:258
Definition: benchmark.h:86
virtual T_e upper_bound()
upper bound specification should be used when upper bound is same for all parameters
Definition: benchmark.h:108
virtual T_e upper_bound()
upper bound specification should be used when upper bound is same for all parameters
Definition: benchmark.h:221
rastrigin_parallel(int dim=2, T_e shift=0.0)
Construct a new rastrigin object.
Definition: benchmark.h:128
virtual T_e upper_bound()
upper bound specification should be used when upper bound is same for all parameters
Definition: benchmark.h:142
Definition: benchmark.h:117
virtual T_e lower_bound()
lower bound specification should be used when lower bound is same for all parameters
Definition: benchmark.h:220
dropwave(int dim=2)
Construct a new dropwave problem.
Definition: benchmark.h:247
Sphere function.
Definition: benchmark.h:35
virtual T_e upper_bound()
upper bound specification should be used when upper bound is same for all parameters
Definition: benchmark.h:312
virtual T_e lower_bound()
lower bound specification should be used when lower bound is same for all parameters
Definition: benchmark.h:141
rastrigin(int dim=2, T_e shift=0.0)
Construct a new rastrigin object.
Definition: benchmark.h:97
Dropwave function referenc : https://www.sfu.ca/~ssurjano/drop.html.
Definition: benchmark.h:235
virtual T_e upper_bound()
upper bound specification should be used when upper bound is same for all parameters
Definition: benchmark.h:49
virtual T_e lower_bound()
lower bound specification should be used when lower bound is same for all parameters
Definition: benchmark.h:176
Definition: benchmark.h:269
virtual T_e lower_bound()
lower bound specification should be used when lower bound is same for all parameters
Definition: benchmark.h:311
virtual T_e upper_bound()
upper bound specification should be used when upper bound is same for all parameters
Definition: benchmark.h:77
Rosenbrock function.
Definition: benchmark.h:62
Definition: benchmark.h:288
virtual T_e lower_bound()
lower bound specification should be used when lower bound is same for all parameters
Definition: benchmark.h:48
Griewank function reference: https://www.sfu.ca/~ssurjano/griewank.html.
Definition: benchmark.h:190
Definition: benchmark.h:151
virtual T_e lower_bound()
lower bound specification should be used when lower bound is same for all parameters
Definition: benchmark.h:257
virtual T_e lower_bound()
lower bound specification should be used when lower bound is same for all parameters
Definition: benchmark.h:107
virtual T_e lower_bound()
lower bound specification should be used when lower bound is same for all parameters
Definition: benchmark.h:76