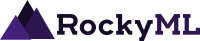 |
RockyML
0.0.1
A High-Performance Scientific Computing Framework
|
5 #ifndef ROCKY_ZAGROS_SYSTEM_GUARD
6 #define ROCKY_ZAGROS_SYSTEM_GUARD
13 #include "spdlog/spdlog.h"
19 template<
typename T_e>
22 virtual T_e objective(T_e* params) = 0;
49 virtual std::string to_string(){
50 return "optimization problem";
58 template<
typename T_e>
64 tbb::enumerable_thread_specific<std::vector<T_e>>* solution_state_;
70 int original_dim()
const{
74 int block_dim()
const{
79 this->main_system_ = main_system;
80 this->original_dim_ = original_dim;
81 this->block_dim_ = block_dim;
82 this->bcd_mask_ = mask;
85 void set_solution_state(tbb::enumerable_thread_specific<std::vector<T_e>>* solution_state){
86 this->solution_state_ = solution_state;
88 virtual T_e objective(T_e* partial){
90 T_e* full_solution = this->solution_state_->local().data();
92 for(
int i=0; i<block_dim_; i++)
93 full_solution[bcd_mask_[i]] = partial[i];
95 return main_system_->objective(full_solution);
virtual T_e lower_bound()
lower bound specification should be used when lower bound is same for all parameters
Definition: system.h:102
a virtual system to implement blocked coordinate descent
Definition: system.h:59
virtual T_e upper_bound(int p_index)
upper bound specification for each parameter should be used if parameters have different upper bounds
Definition: system.h:48
virtual T_e lower_bound(int p_index)
lower bound specification for each parameter should be used if parameters have different lower bounds
Definition: system.h:109
virtual T_e upper_bound(int p_index)
upper bound specification for each parameter should be used if parameters have different upper bounds
Definition: system.h:122
virtual T_e upper_bound()
upper bound specification should be used when upper bound is same for all parameters
Definition: system.h:41
virtual T_e lower_bound()
lower bound specification should be used when lower bound is same for all parameters
Definition: system.h:28
virtual T_e upper_bound()
upper bound specification should be used when upper bound is same for all parameters
Definition: system.h:115
virtual T_e lower_bound(int p_index)
lower bound specification for each parameter should be used if parameters have different lower bounds
Definition: system.h:35